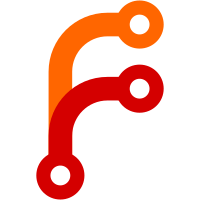
* initial version of the advanced tab * added registered nodes * added fine grain open id connect configuration * added open id connect compatibility section * added advanced section * added backend * fixed type * renamed 'advanced' to advancedtab to prevent strange add of '/index.js' by snowpack * fixed storybook stories * change '_' to '-' because '_' is also used * fix spacing buttons * stop passing the form * cypress test for advanced tab * more tests * saml section * changed to use NumberInput * added authetnication flow override * fixed merge error * updated text and added link to settings tab * fixed test * added filter on flows and better reset * added now mandetory error handler * added sorting * Revert "changed to use NumberInput" This reverts commit 7829f2656ae8fc8ed4a4a6b1c4b1961241a09d8e. * allow users to put empty string as value * already on detail page after save * fixed merge error
50 lines
1.2 KiB
TypeScript
50 lines
1.2 KiB
TypeScript
import React, { ReactNode } from "react";
|
|
import { Meta } from "@storybook/react";
|
|
|
|
import { DataLoader } from "../components/data-loader/DataLoader";
|
|
|
|
export default {
|
|
title: "Data Loader",
|
|
component: DataLoader,
|
|
} as Meta;
|
|
|
|
type Post = {
|
|
title: string;
|
|
body: string;
|
|
};
|
|
|
|
export const loadPosts = () => {
|
|
const PostLoader = (props: { url: string; children: ReactNode }) => {
|
|
const loader = async () => {
|
|
const wait = (ms: number, value: Post) =>
|
|
new Promise((resolve) => setTimeout(resolve, ms, value));
|
|
return await fetch(props.url)
|
|
.then((res) => res.json())
|
|
.then((value) => wait(3000, value));
|
|
};
|
|
return <DataLoader loader={loader}>{props.children}</DataLoader>;
|
|
};
|
|
|
|
return (
|
|
<PostLoader url="https://jsonplaceholder.typicode.com/posts">
|
|
{(posts: Post[]) => (
|
|
<table>
|
|
<thead>
|
|
<tr>
|
|
<th>Name</th>
|
|
<th>Description</th>
|
|
</tr>
|
|
</thead>
|
|
<tbody>
|
|
{posts.map((post, i) => (
|
|
<tr key={i}>
|
|
<td>{post.title}</td>
|
|
<td>{post.body}</td>
|
|
</tr>
|
|
))}
|
|
</tbody>
|
|
</table>
|
|
)}
|
|
</PostLoader>
|
|
);
|
|
};
|