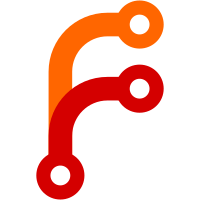
* generate provider form with the dynmic components Added a FileComponent and removed the need for custom views * fixed merge error * fixed tests * Update src/realm-settings/keys/key-providers/KeyProviderForm.tsx Co-authored-by: Jon Koops <jonkoops@gmail.com> Co-authored-by: Jon Koops <jonkoops@gmail.com>
53 lines
1.6 KiB
TypeScript
53 lines
1.6 KiB
TypeScript
import type { FunctionComponent } from "react";
|
|
|
|
import type { ConfigPropertyRepresentation } from "@keycloak/keycloak-admin-client/lib/defs/authenticatorConfigInfoRepresentation";
|
|
import { StringComponent } from "./StringComponent";
|
|
import { BooleanComponent } from "./BooleanComponent";
|
|
import { ListComponent } from "./ListComponent";
|
|
import { RoleComponent } from "./RoleComponent";
|
|
import { MapComponent } from "./MapComponent";
|
|
import { ScriptComponent } from "./ScriptComponent";
|
|
import { ClientSelectComponent } from "./ClientSelectComponent";
|
|
import { MultiValuedStringComponent } from "./MultivaluedStringComponent";
|
|
import { MultiValuedListComponent } from "./MultivaluedListComponent";
|
|
import { GroupComponent } from "./GroupComponent";
|
|
import { FileComponent } from "./FileComponent";
|
|
|
|
export type ComponentProps = Omit<ConfigPropertyRepresentation, "type"> & {
|
|
isDisabled?: boolean;
|
|
};
|
|
|
|
const ComponentTypes = [
|
|
"String",
|
|
"boolean",
|
|
"List",
|
|
"Role",
|
|
"Script",
|
|
"Map",
|
|
"Group",
|
|
"MultivaluedList",
|
|
"ClientList",
|
|
"MultivaluedString",
|
|
"File",
|
|
] as const;
|
|
|
|
export type Components = typeof ComponentTypes[number];
|
|
|
|
export const COMPONENTS: {
|
|
[index in Components]: FunctionComponent<ComponentProps>;
|
|
} = {
|
|
String: StringComponent,
|
|
boolean: BooleanComponent,
|
|
List: ListComponent,
|
|
Role: RoleComponent,
|
|
Script: ScriptComponent,
|
|
Map: MapComponent,
|
|
Group: GroupComponent,
|
|
ClientList: ClientSelectComponent,
|
|
MultivaluedList: MultiValuedListComponent,
|
|
MultivaluedString: MultiValuedStringComponent,
|
|
File: FileComponent,
|
|
} as const;
|
|
|
|
export const isValidComponentType = (value: string): value is Components =>
|
|
value in COMPONENTS;
|