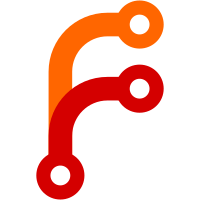
* initial version of the advanced tab * added registered nodes * added fine grain open id connect configuration * added open id connect compatibility section * added advanced section * added backend * fixed type * renamed 'advanced' to advancedtab to prevent strange add of '/index.js' by snowpack * fixed storybook stories * change '_' to '-' because '_' is also used * fix spacing buttons * stop passing the form * cypress test for advanced tab * more tests * saml section * changed to use NumberInput * added authetnication flow override * fixed merge error * updated text and added link to settings tab * fixed test * added filter on flows and better reset * added now mandetory error handler * added sorting * Revert "changed to use NumberInput" This reverts commit 7829f2656ae8fc8ed4a4a6b1c4b1961241a09d8e. * allow users to put empty string as value * already on detail page after save * fixed merge error
63 lines
1.9 KiB
TypeScript
63 lines
1.9 KiB
TypeScript
import React from "react";
|
|
import { FormGroup, TextInput, ValidatedOptions } from "@patternfly/react-core";
|
|
import { useFormContext } from "react-hook-form";
|
|
import { useTranslation } from "react-i18next";
|
|
|
|
import { FormAccess } from "../components/form-access/FormAccess";
|
|
import { ClientForm } from "./ClientDetails";
|
|
|
|
export const ClientDescription = () => {
|
|
const { t } = useTranslation("clients");
|
|
const { register, errors } = useFormContext<ClientForm>();
|
|
return (
|
|
<FormAccess role="manage-clients" unWrap>
|
|
<FormGroup
|
|
label={t("clientID")}
|
|
fieldId="kc-client-id"
|
|
helperTextInvalid={t("common:required")}
|
|
validated={
|
|
errors.clientId ? ValidatedOptions.error : ValidatedOptions.default
|
|
}
|
|
isRequired
|
|
>
|
|
<TextInput
|
|
ref={register({ required: true })}
|
|
type="text"
|
|
id="kc-client-id"
|
|
name="clientId"
|
|
validated={
|
|
errors.clientId ? ValidatedOptions.error : ValidatedOptions.default
|
|
}
|
|
/>
|
|
</FormGroup>
|
|
<FormGroup label={t("common:name")} fieldId="kc-name">
|
|
<TextInput ref={register()} type="text" id="kc-name" name="name" />
|
|
</FormGroup>
|
|
<FormGroup
|
|
label={t("common:description")}
|
|
fieldId="kc-description"
|
|
validated={
|
|
errors.description ? ValidatedOptions.error : ValidatedOptions.default
|
|
}
|
|
helperTextInvalid={errors.description?.message}
|
|
>
|
|
<TextInput
|
|
ref={register({
|
|
maxLength: {
|
|
value: 255,
|
|
message: t("common:maxLength", { length: 255 }),
|
|
},
|
|
})}
|
|
type="text"
|
|
id="kc-description"
|
|
name="description"
|
|
validated={
|
|
errors.description
|
|
? ValidatedOptions.error
|
|
: ValidatedOptions.default
|
|
}
|
|
/>
|
|
</FormGroup>
|
|
</FormAccess>
|
|
);
|
|
};
|