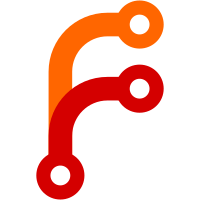
* Add credential reset modal * Add i18n labels * Refactor to align with marvelapp mockup TODO: tests * Add e2e tests * Implement code review change requests Add menuAppendTo to CredentialResetActionMultiSelect -> Select component Add optional menuAppendTo prop to TimeSelectorComponent Refactor CredentialsPage constructor
86 lines
2.1 KiB
TypeScript
86 lines
2.1 KiB
TypeScript
export default class CredentialsPage {
|
|
private readonly credentialsTab = "credentials";
|
|
private readonly emptyStatePasswordBtn = "no-credentials-empty-action";
|
|
private readonly emptyStateResetBtn = "credential-reset-empty-action";
|
|
private readonly resetBtn = "credentialResetBtn";
|
|
private readonly setPasswordBtn = "setPasswordBtn";
|
|
private readonly credentialResetModal = "credential-reset-modal";
|
|
private readonly resetModalActionsToggleBtn =
|
|
"[data-testid=credential-reset-modal] #actions";
|
|
private readonly passwordField =
|
|
".kc-password > .pf-c-input-group > .pf-c-form-control";
|
|
private readonly passwordConfirmationField =
|
|
".kc-passwordConfirmation > .pf-c-input-group > .pf-c-form-control";
|
|
private readonly resetActions = [
|
|
"VERIFY_EMAIL-option",
|
|
"UPDATE_PROFILE-option",
|
|
"CONFIGURE_TOTP-option",
|
|
"UPDATE_PASSWORD-option",
|
|
"terms_and_conditions-option",
|
|
];
|
|
private readonly confirmationButton = "okBtn";
|
|
|
|
goToCredentialsTab() {
|
|
cy.findByTestId(this.credentialsTab).click();
|
|
|
|
return this;
|
|
}
|
|
clickEmptyStatePasswordBtn() {
|
|
cy.findByTestId(this.emptyStatePasswordBtn).click();
|
|
|
|
return this;
|
|
}
|
|
|
|
clickEmptyStateResetBtn() {
|
|
cy.findByTestId(this.emptyStateResetBtn).click();
|
|
|
|
return this;
|
|
}
|
|
|
|
clickResetBtn() {
|
|
cy.findByTestId(this.resetBtn).click();
|
|
|
|
return this;
|
|
}
|
|
|
|
clickResetModalActionsToggleBtn() {
|
|
cy.get(this.resetModalActionsToggleBtn).click();
|
|
|
|
return this;
|
|
}
|
|
|
|
clickResetModalAction(index: number) {
|
|
cy.findByTestId(this.resetActions[index]).click();
|
|
|
|
return this;
|
|
}
|
|
|
|
clickConfirmationBtn() {
|
|
cy.findByTestId(this.confirmationButton).dblclick();
|
|
|
|
return this;
|
|
}
|
|
|
|
fillPasswordForm() {
|
|
cy.get(this.passwordField).type("test");
|
|
cy.get(this.passwordConfirmationField).type("test");
|
|
|
|
return this;
|
|
}
|
|
|
|
fillResetCredentialForm() {
|
|
cy.findByTestId(this.credentialResetModal);
|
|
this.clickResetModalActionsToggleBtn()
|
|
.clickResetModalAction(2)
|
|
.clickResetModalAction(3)
|
|
.clickConfirmationBtn();
|
|
|
|
return this;
|
|
}
|
|
|
|
clickSetPasswordBtn() {
|
|
cy.findByTestId(this.setPasswordBtn).click();
|
|
|
|
return this;
|
|
}
|
|
}
|