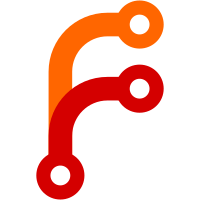
Closes #21345 Closes #21344 Signed-off-by: Jon Koops <jonkoops@gmail.com> Co-authored-by: Erik Jan de Wit <erikjan.dewit@gmail.com> Co-authored-by: Mark Franceschelli <mfrances@redhat.com> Co-authored-by: Hynek Mlnařík <hmlnarik@redhat.com> Co-authored-by: Agnieszka Gancarczyk <agancarc@redhat.com>
74 lines
1.7 KiB
TypeScript
74 lines
1.7 KiB
TypeScript
import {
|
|
FormHelperText,
|
|
HelperText,
|
|
HelperTextItem,
|
|
TextInput,
|
|
TextInputProps,
|
|
ValidatedOptions,
|
|
} from "@patternfly/react-core";
|
|
import { ReactNode } from "react";
|
|
import {
|
|
FieldPath,
|
|
FieldValues,
|
|
PathValue,
|
|
UseControllerProps,
|
|
useController,
|
|
} from "react-hook-form";
|
|
|
|
import { FormLabel } from "./FormLabel";
|
|
|
|
export type TextControlProps<
|
|
T extends FieldValues,
|
|
P extends FieldPath<T> = FieldPath<T>,
|
|
> = UseControllerProps<T, P> &
|
|
Omit<TextInputProps, "name" | "isRequired" | "required"> & {
|
|
label: string;
|
|
labelIcon?: string | ReactNode;
|
|
isDisabled?: boolean;
|
|
helperText?: string;
|
|
};
|
|
|
|
export const TextControl = <
|
|
T extends FieldValues,
|
|
P extends FieldPath<T> = FieldPath<T>,
|
|
>(
|
|
props: TextControlProps<T, P>,
|
|
) => {
|
|
const { labelIcon, ...rest } = props;
|
|
const required = !!props.rules?.required;
|
|
const defaultValue = props.defaultValue ?? ("" as PathValue<T, P>);
|
|
|
|
const { field, fieldState } = useController({
|
|
...props,
|
|
defaultValue,
|
|
});
|
|
|
|
return (
|
|
<FormLabel
|
|
name={props.name}
|
|
label={props.label}
|
|
labelIcon={labelIcon}
|
|
isRequired={required}
|
|
error={fieldState.error}
|
|
>
|
|
<TextInput
|
|
isRequired={required}
|
|
id={props.name}
|
|
data-testid={props.name}
|
|
validated={
|
|
fieldState.error ? ValidatedOptions.error : ValidatedOptions.default
|
|
}
|
|
isDisabled={props.isDisabled}
|
|
{...rest}
|
|
{...field}
|
|
/>
|
|
{props.helperText && (
|
|
<FormHelperText>
|
|
<HelperText>
|
|
<HelperTextItem>{props.helperText}</HelperTextItem>
|
|
</HelperText>
|
|
</FormHelperText>
|
|
)}
|
|
</FormLabel>
|
|
);
|
|
};
|