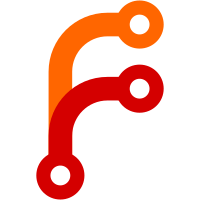
* initial version Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com> * better init Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com> * added more components Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com> * moved to public Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com> * use environment var to enter library mode Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com> * added export field Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com> --------- Signed-off-by: Erik Jan de Wit <erikjan.dewit@gmail.com>
69 lines
1.7 KiB
TypeScript
69 lines
1.7 KiB
TypeScript
import { CallOptions } from "./api/methods";
|
|
import { Links, parseLinks } from "./api/parse-links";
|
|
import { parseResponse } from "./api/parse-response";
|
|
import { Permission, Resource, Scope } from "./api/representations";
|
|
import { request } from "./api/request";
|
|
import { KeycloakContext } from "./root/KeycloakContext";
|
|
|
|
export const fetchResources = async (
|
|
{ signal, context }: CallOptions,
|
|
requestParams: Record<string, string>,
|
|
shared: boolean | undefined = false,
|
|
): Promise<{ data: Resource[]; links: Links }> => {
|
|
const response = await request(
|
|
`/resources${shared ? "/shared-with-me?" : "?"}`,
|
|
context,
|
|
{ searchParams: shared ? requestParams : undefined, signal },
|
|
);
|
|
|
|
let links: Links;
|
|
|
|
try {
|
|
links = parseLinks(response);
|
|
} catch (error) {
|
|
links = {};
|
|
}
|
|
|
|
return {
|
|
data: checkResponse(await response.json()),
|
|
links,
|
|
};
|
|
};
|
|
|
|
export const fetchPermission = async (
|
|
{ signal, context }: CallOptions,
|
|
resourceId: string,
|
|
): Promise<Permission[]> => {
|
|
const response = await request(
|
|
`/resources/${resourceId}/permissions`,
|
|
context,
|
|
{ signal },
|
|
);
|
|
return parseResponse<Permission[]>(response);
|
|
};
|
|
|
|
export const updateRequest = (
|
|
context: KeycloakContext,
|
|
resourceId: string,
|
|
username: string,
|
|
scopes: Scope[] | string[],
|
|
) =>
|
|
request(`/resources/${resourceId}/permissions`, context, {
|
|
method: "PUT",
|
|
body: [{ username, scopes }],
|
|
});
|
|
|
|
export const updatePermissions = (
|
|
context: KeycloakContext,
|
|
resourceId: string,
|
|
permissions: Permission[],
|
|
) =>
|
|
request(`/resources/${resourceId}/permissions`, context, {
|
|
method: "PUT",
|
|
body: permissions,
|
|
});
|
|
|
|
function checkResponse<T>(response: T) {
|
|
if (!response) throw new Error("Could not fetch");
|
|
return response;
|
|
}
|